The time is {% now "jS F Y H:i" %}
Hello, {{ request.user.email }}!
{% else %}You are not logged in.
{% endif %} ``` Or in Python: ```python if request.user: print(f"Hello, {request.user.email}!") else: print("You are not logged in.") ``` ## Restricting views Use the `AuthViewMixin` to restrict views to logged in users, admin users, or custom logic. ```python from plain.auth.views import AuthViewMixin from plain.exceptions import PermissionDenied from plain.views import View class LoggedInView(AuthViewMixin, View): login_required = True class AdminOnlyView(AuthViewMixin, View): login_required = True admin_required = True class CustomPermissionView(AuthViewMixin, View): def check_auth(self): super().check_auth() if not self.request.user.is_special: raise PermissionDenied("You're not special!") ```Foo is enabled for you!
{% else %}Foo is disabled for you.
{% endif %} ``` Or in Python: ```python import flags print(flags.FooEnabled(user).value) ``` ## Installation ```python INSTALLED_PACKAGES = [ ... "plain.flags", ] ``` Create a `flags.py` at the top of your `app` (or point `settings.FLAGS_MODULE` to a different location). ## Advanced usage Ultimately you can do whatever you want inside of `get_key` and `get_value`. ```python class OrganizationFeature(Flag): url_param_name = "" def __init__(self, request=None, organization=None): # Both of these are optional, but will usually both be given self.request = request self.organization = organization def get_key(self): if ( self.url_param_name and self.request and self.url_param_name in self.request.query_params ): return None if not self.organization: # Don't save the flag result for PRs without an organization return None return self.organization def get_value(self): if self.url_param_name and self.request: if self.request.query_params.get(self.url_param_name) == "1": return True if self.request.query_params.get(self.url_param_name) == "0": return False if not self.organization: return False # All organizations will start with False, # and I'll override in the DB for the ones that should be True return False class AIEnabled(OrganizationFeature): pass ```The time is {% now "jS F Y H:i" %}
State: {{ pullrequest.state }}
{% if pullrequest.state == "open" %}{{ oauth_error }}
{% endblock %} ``` 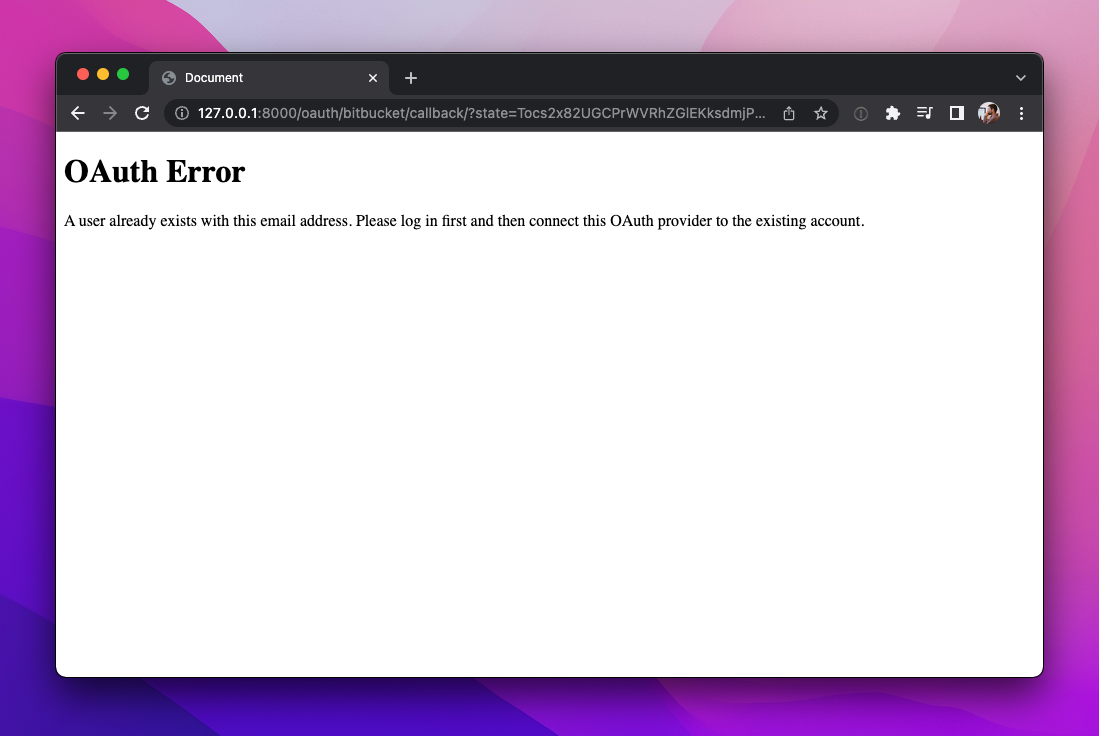 ### Connecting and disconnecting OAuth accounts To connect and disconnect OAuth accounts, you can add a series of forms to a user/profile settings page. Here's an very basic example: ```html {% extends "base.html" %} {% block content %} Hello {{ request.user }}!